Concurrency with Modern C++ is a journey through current and upcoming concurrency in C++.
- C++11 and C++14 have the basic building blocks for creating concurrent or parallel programs.
- With C++17 we got the parallel algorithms of the Standard Template Library (STL). That means, most of the algorithms of the STL can be executed sequential, parallel, or vectorized.
- The concurrency story in C++ goes on. With C++20 we can hope for extended futures, coroutines, transactions, and more.
How to Get it?
Use the link: Leanpub.com: Concurrency with Modern C++
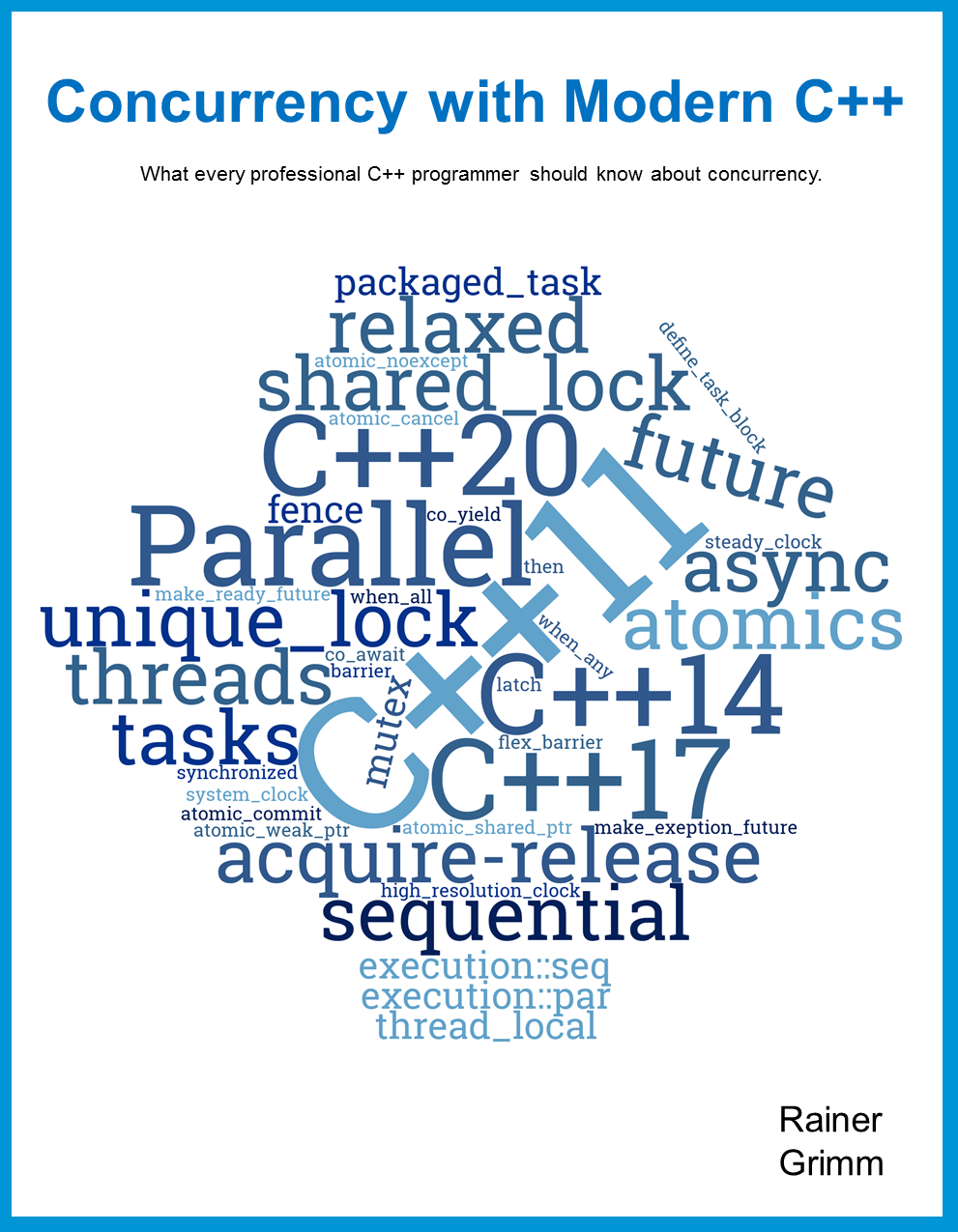
What's Inside?
This book explains you the details to concurrency in modern C++ and gives you, in addition, more than 100 running code examples. Therefore you can combine the theory with the practices and get the most of it.
Because this book is about concurrency, I present a lot of pitfalls and show you how to overcome them.
Give me the Details!
If you are curious and you should be, here are the details to the more than 300 pages:
- Introduction
- Conventions
- Source Code
- How you should read the book?
- Personal Notes
- Acknowledgements
- About Me
- My Special Circumstances
- A Quick Overview
- Concurrency with Modern C++
- C++11 and C++14: The foundation
- Memory Model
- Multithreading
- Case Studies
- Calculating the Sum of a Vector
- Thread-Safe Initialisation of a Singleton
- Ongoing Optimisation with CppMem
- C++17: Parallel Algorithms of the Standard Template Library
- Execution Policy
- New Algorithms
- C++20: The Concurrent Future
- Atomic Smart Pointers
- Extended futures
- Latches and Barriers
- Coroutines
- Transactional Memory
- Task Blocks
- Challenges
- Best Practices
- Time Library
- Glossary
- The Details
- Memory Model
- The Contract
- The Foundation
- The Challenges
- Atomics
- Strong versus Weak Memory Model
- The Atomic Flag
- The Class Template
std::atomic
- User Defined Atomics
- All Atomic Operations
- Free Atomic Functions
std::shared_ptr
- The Synchronisation and Ordering Constraints
- The six variants of the C++ memory model
- Sequential Consistency
- Acquire-Release Semantic
- std::memory_order_consume
- Relaxed Semantic
- Fences
- Fences as Memory Barriers
- The three Fences
- Acquire-Release Fences
- Synchronisation with Atomic Variables or Fences
- Multithreading
- Threads
- Creation
- Lifetime
- Arguments
- Methods
- Shared Data
- Mutexes
- Locks
- Thread-safe Initialisation
- Thread-Local Data
- Condition Variables
- The Wait Workflow
- Lost Wakeup and Spurious Wakeup
- Tasks
- Threads versus Tasks
- std::async
- std::packaged_task
- std::promise and std::future
- Case Studies
- Calculating the Sum of a Vector
- Single Threaded addition of a Vector
- Multithreaded Summation with a Shared Variable
- Thread-Local Summation
- Summation of a Vector: The Conclusion
- Thread-Safe Initialisation of a Singleton
- Double-Checked Locking Pattern
- My Strategy
- Thread-Safe Meyers Singleton
std::call_once
with the std::once_flag
- Atomics
- Performance Numbers of the various Thread-Safe Singleton Implementations
- Ongoing Optimisation with CppMem
- CppMem - An Overview
- CppMem: Non-Atomic Variables
- CppMem: Locks
- CppMem: Atomics with Sequential Consistency
- CppMem: Atomics with Acquire-Release Semantic
- CppMem: Atomics with Non-atomics
- CppMem: Atomics with Relaxed Semantic
- Parallel Algorithms of the Standard Template Library
- Execution Policies
- Algorithms
- The Future: C++20
- Atomic Smart Pointers
- A thread-safe singly linked list
- Extended Futures
std::future
std::async
, std::packaged_task
, and std::promise
- Creating new Futures
- Latches and Barriers
std::latch
std::barrier
std::flex_barrier
- Coroutines
- A Generator Function
- Details
- Transactional Memory
- ACI(D)
- Synchronized and Atomic Blocks
transaction_safe
versus transaction_unsafe
Code
- Task Blocks
- Fork and Join
define_task_block
versus define_task_block_restore_thread
- The Interface
- The Scheduler
- Further Information
- Challenges
- ABA
- Blocking Issues
- Breaking of Program Invariants
- Data Races
- False Sharing
- Lifetime Issues of Variables
- Moving Threads
- Deadlocks
- Race Conditions
- Best Practices
- General
- Code Reviews
- Minimise Data Sharing of mutable data
- Minimise Waiting
- Prefer Immutable Data
- Look for the Right Abstraction
- Use Static Code Analysis Tools
- Memory Model
- Don’t use volatile for synchronisation
- Don’t program Lock Free
- If you program Lock-Free, use well established patterns
- Don’t build your own abstraction, use guarantees of the language
- Multithreading
- Threads
- Data Sharing
- Condition Variables
- Promises and Futures
- The Time Library
- The Interplay of Time Point, Time Duration, and Clock
- Time Point
- From Time Point to Calendar Time
- Cross the valid Time Range
- Time Duration
- Clocks
- Accuracy and Steadiness
- Epoch
- Sleep and Wait
- Glossary
- ACID
- Callable Unit
- Concurrency
- Critical Section
- Function Objects
- Lambda Functions
- Lock-free
- Lost Wakeup
- Modification Order
- Monad
- Non-blocking
- Parallelism
- Predicate
- RAII
- Sequential Consistency
- Sequence Point
- Spurious Wakeup
- Thread
- Total order
- volatile
- wait-free
- Index
Modernes C++,

Thanks a lot to my Patreon Supporter: Eric Pederson.
Get your e-book at leanpub:
The C++ Standard Library
|
|
Concurrency with Modern C++
|
|
Get Both as one Bundle
|
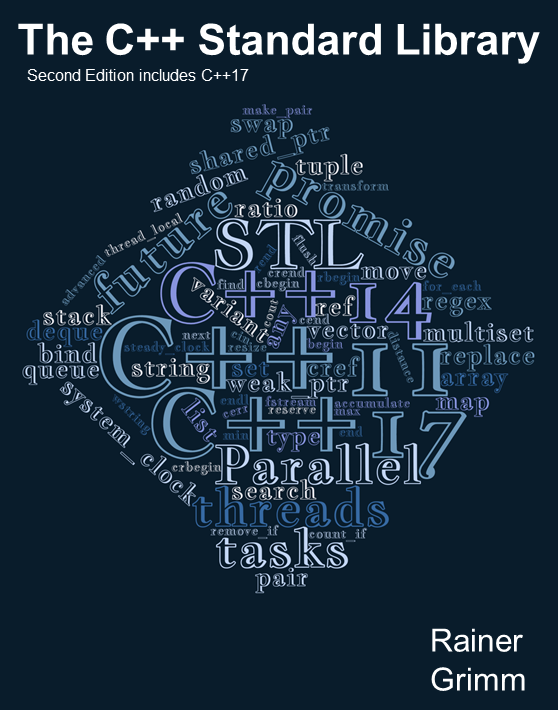 |
|
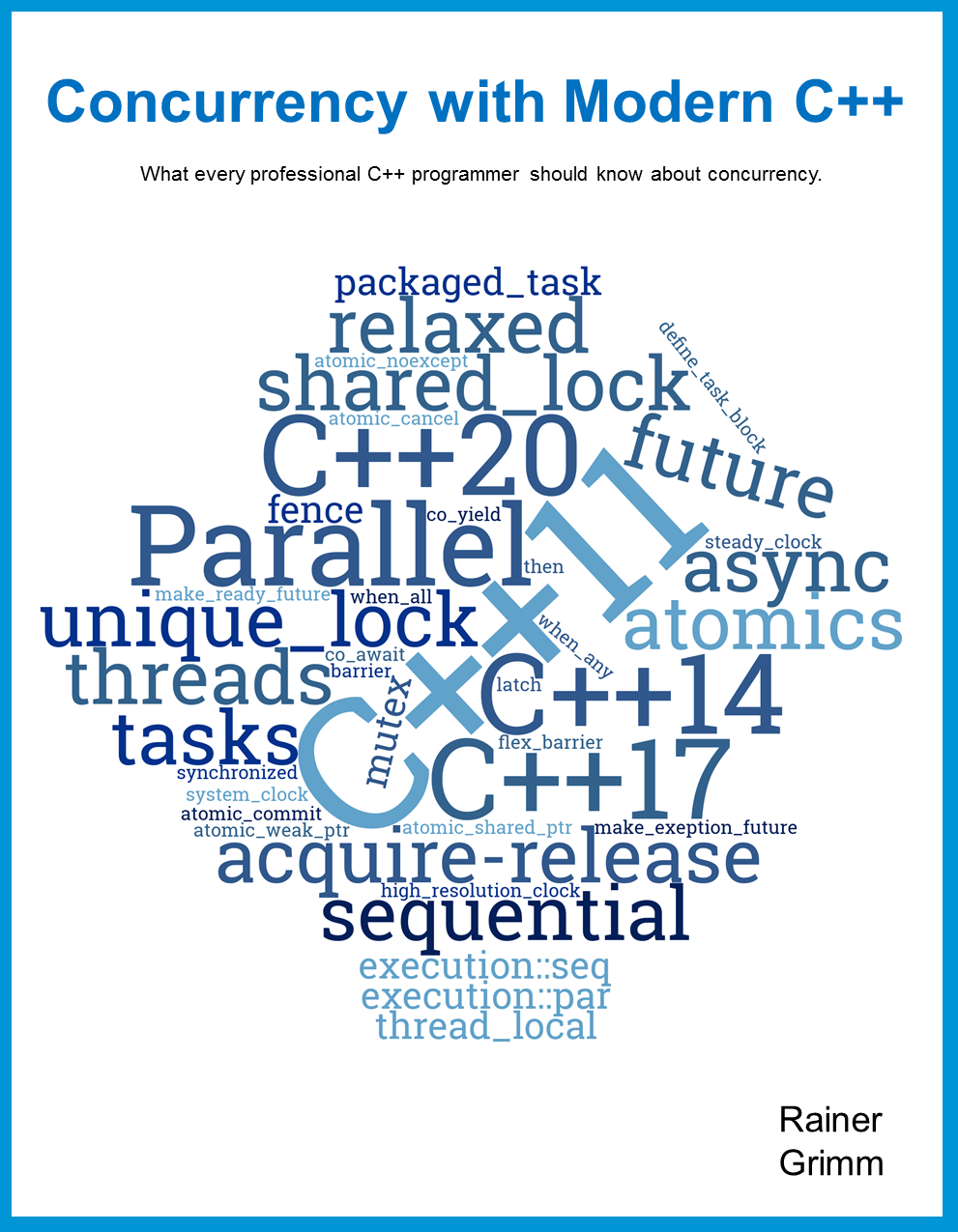 |
|
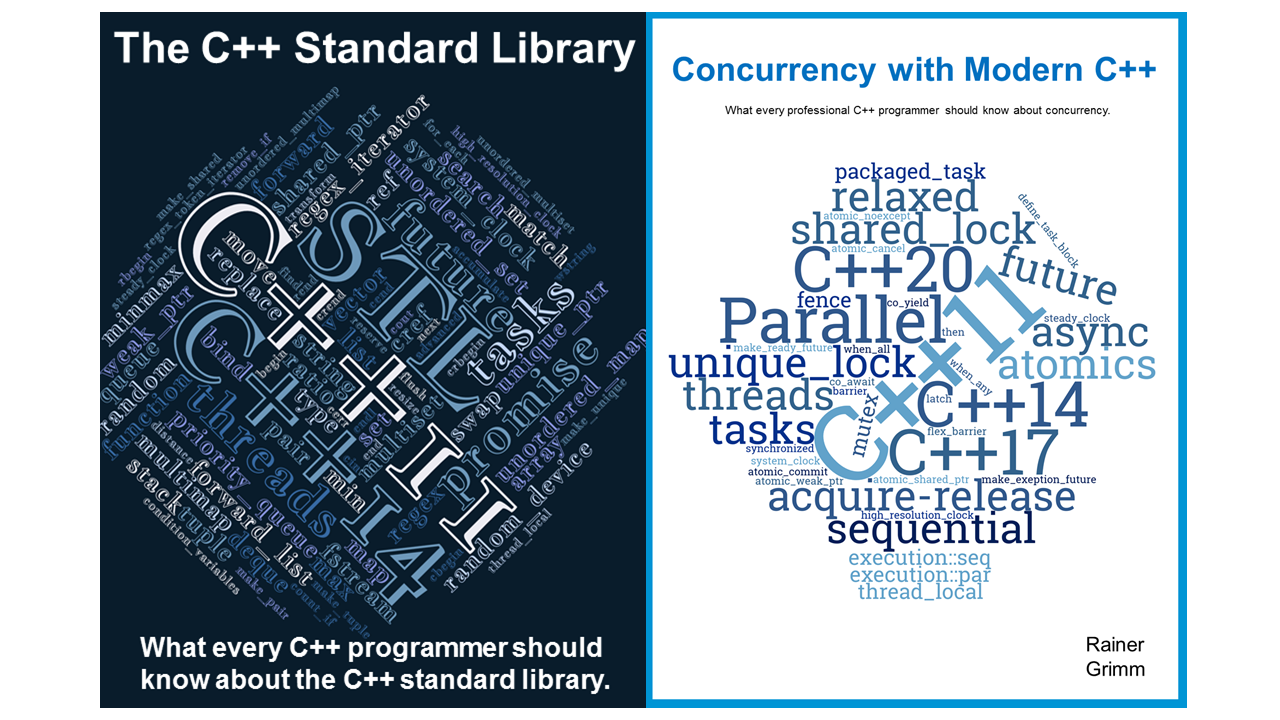 |
With C++11 and C++14 we got a lot of new C++ libraries. In addition, the existing ones are greatly improved. The key idea of my book is to give you the necessary information to the current C++ libraries in about 200 pages. |
|
C++11 is the first C++ standard that deals with concurrency. The story goes on with C++17 and will continue with C++20.
I'll give you a detailed insight in the current and the upcoming concurrency in C++. This insight includes the theory and a lot of practice with more the 100 source files.
|
|
Get my books "The C++ Standard Library" and "Concurrency with Modern C++" in a bundle.
In sum, you get more than 500 pages full of modern C++ and more than 100 source files presenting concurrency in practice.
|
Weiterlesen...